Fuzzy Farmers
Engine: Unreal Engine 5
Intended Platform: PC
Approximate Date of Development: October 20th, 2024
Genre: Simulation
What is it?
Fuzzy Farmers is a game that I initially created for my GAM 303 course, which I took at the start of 2024. The course focuses on the fundamental ideas of game development and design, and teaches basic skills using blueprints only.
My goal after the course was to start from the beginning and apply the knowledge I gained as I took more courses and learned more about game development and Unreal Engine. Additionally, the first version of the game was in Unreal Engine 4, and I wanted to get more experience with Unreal Engine 5.
The resulting product displayed here is the most recent version of the prototype. It shows off main gameplay features and a general feel for the world. At this point the gameplay is brief, but the foundation has been laid to continue development.
Description of Gameplay
In this prototype, the player’s goal is to capture as many fuzzies as possible within 5 minutes. The player can find fuzzies by listening for sound cues and watching for visual cues. When the player approaches a location that they think might have a fuzzy inside, they press and hold the E key to search. Once they’ve finished searching, if a fuzzy is inside, it will pop out and start running away from the player. The player then chases the fuzzy, presses E to catch it, and their score is increased.
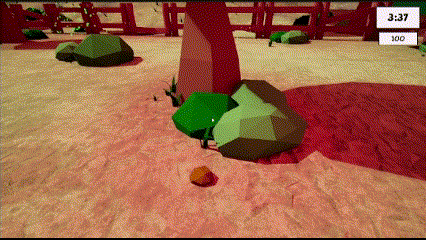
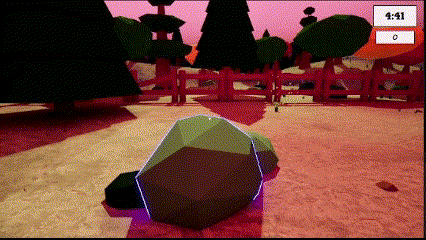
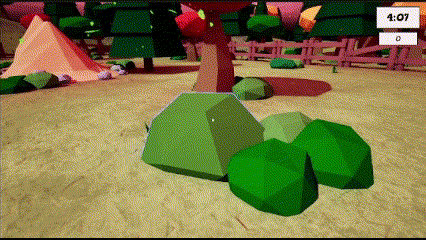
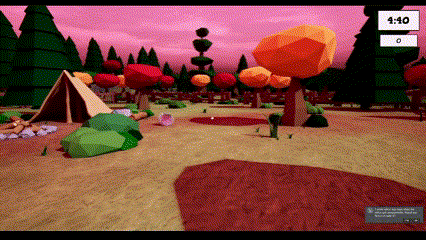
Fuzzy
One of the main features of the game, if not the most important, is the Fuzzy. It’s a simple sphere static mesh with a groom asset attached. The gif below shows what the fuzzy looks like before dynamic materials are applied and modified. The default colors are intentionally clashing so that if there is an issue with the materials, it’s easy to see when the game is simulated.
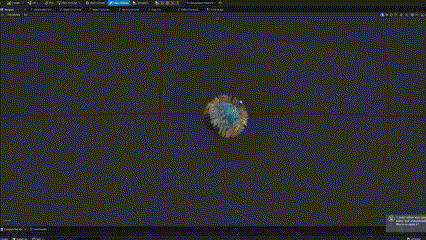
Before creating the groom asset, I experimented with making the Fuzzy out of larger hair pieces, using regular meshes and inverse kinematics. But this was difficult and didn’t achieve the look I was going for.
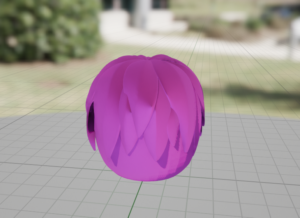
So I decided to learn how to make hair in blender! It turned out to be much more straightforward than I had anticipated. I went through many different versions of the hair, but I knew I wanted a “clumpy” style to eventually resemble the low-poly style of the environment.
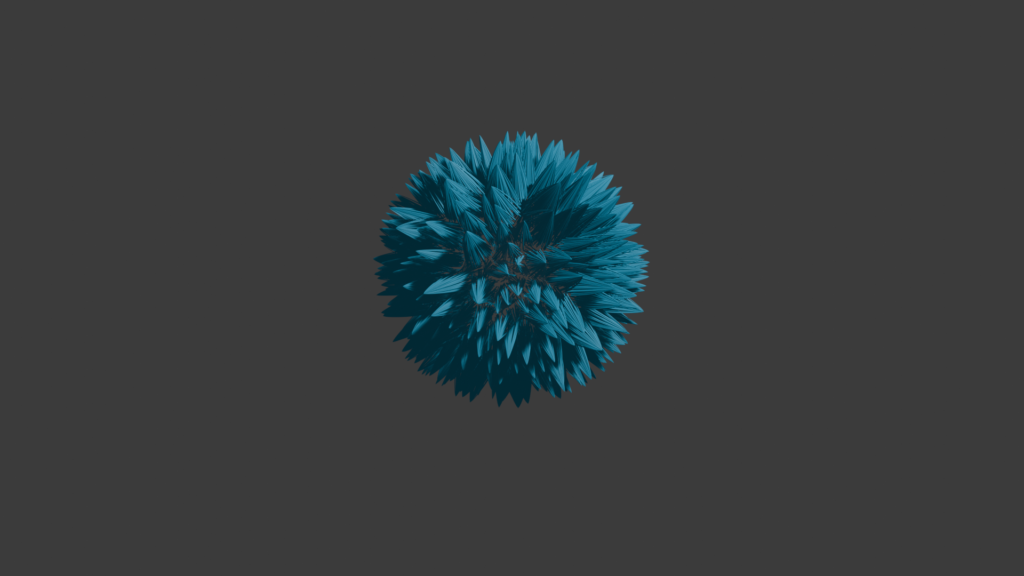
AFuzzy::AFuzzy()
{
PrimaryActorTick.bCanEverTick = true;
fuzzyMesh = CreateDefaultSubobject<USkeletalMeshComponent>(TEXT("FuzzyMesh"));
RootComponent = fuzzyMesh;
captureCollision = CreateDefaultSubobject<USphereComponent>(TEXT("CaptureCollision"));
captureCollision->SetupAttachment(fuzzyMesh);
captureCollision->SetCollisionEnabled(ECollisionEnabled::NoCollision);
playerDistanceCollision = CreateDefaultSubobject<USphereComponent>(TEXT("PlayerDistanceCollision"));
playerDistanceCollision->SetupAttachment(fuzzyMesh);
AIControllerClass = AFuzzyAIController::StaticClass();
}
// Called when the game starts or when spawned
void AFuzzy::BeginPlay()
{
Super::BeginPlay();
NavSys = UNavigationSystemV1::GetCurrent(GetWorld());
PlayerCharacter = UGameplayStatics::GetPlayerPawn(GetWorld(), 0);
dmiMat = UMaterialInstanceDynamic::Create(fuzzyMat, this);
if (dmiMat != nullptr)
{
fuzzyMesh->SetMaterial(0, dmiMat);
dmiMat->SetVectorParameterValue("FuzzyColor", fuzzyColor);
}
if (playerDistanceCollision != nullptr)
{
//GEngine->AddOnScreenDebugMessage(-1, 5, FColor::Red, "not null");
}
else
{
//GEngine->AddOnScreenDebugMessage(-1, 5, FColor::Red, "null");
}
LaunchTowardsPlayer();
}
Above is some of the code that modifies the materials for the Fuzzy.
Spawnpoints
Spawnpoints are the locations/objects that Fuzzies can spawn from. There are four different options:
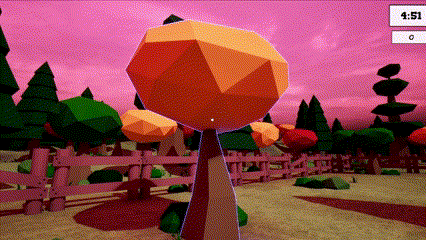
Trees
Trees produce a burst of leaves from the top section. To add variation to the game, the color of the leaves can be either orange, red, or green. This value is determined at the start of the game, before Fuzzies are spawned into the level.
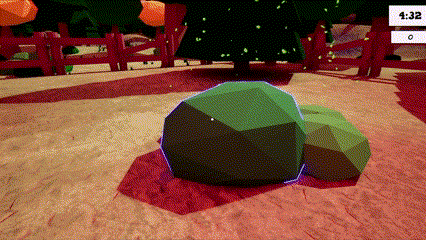
Bushes
Bushes produce a burst of leaves from the top when there is a Fuzzy inside. The color of the leaves and the mesh are randomized before the game starts.
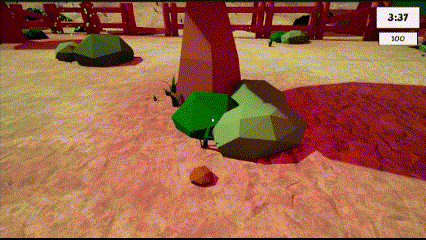
Dirt
Dirt spots are small brown spots scattered throughout the level. They produce small dirt particles periodically to indicate the presence of a Fuzzy.
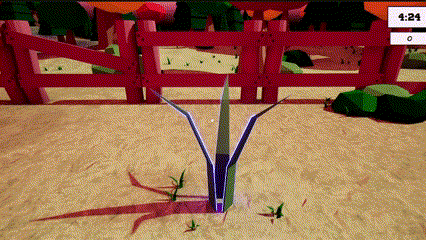
Grass
Grass clusters are scattered throughout the level, similarly to dirt. Initially there was an issue with the Fuzzies being hard to detect if they were hiding inside these. As a result, I increased the scale of the grass spawnpoints that contain Fuzzies.
The Code
Each spawnpoint is a child of the parent class, Spawnpoint Parent. Because each spawnpoint overall has the same intended purpose and functionalities, it made the most sense to build one class, and make any modifications necessary in the child class. This was a great opportunity for me to really understand the principles of Object Oriented Programming.
Reveal Code
ASpawnpointParent::ASpawnpointParent()
{
// Set this actor to call Tick() every frame. You can turn this off to improve performance if you don't need it.
PrimaryActorTick.bCanEverTick = true;
// Collision for detecting when the player's center of the screen is hovering over a spawnpoint.
rayCastCollision = CreateDefaultSubobject<USphereComponent>(TEXT("RaycastCollision"));
rayCastCollision->OnComponentHit.AddDynamic(this, &ASpawnpointParent::OnHit);
// Sets the raycast collision as the root component
RootComponent = rayCastCollision;
// Collision for the player. To avoid the player getting too close and being awkardly on top of the spawnpoint
playerCollision = CreateDefaultSubobject<UBoxComponent>(TEXT("PlayerCollision"));
playerCollision->SetupAttachment(rayCastCollision);
// Static mesh
mesh = CreateDefaultSubobject<UStaticMeshComponent>(TEXT("BushMesh"));
mesh->SetupAttachment(rayCastCollision);
// Arrow component for the direction of the leaf/dirt burst.
arrowcomponent = CreateDefaultSubobject<UArrowComponent>(TEXT("ArrowComponent"));
arrowcomponent->SetupAttachment(mesh);
// Spring arm component for where the fuzzy should spawn.
springArmComponent = CreateDefaultSubobject<USpringArmComponent>(TEXT("SpringArmComponent"));
springArmComponent->SetupAttachment(mesh);
// Default containsFuzzy should be false
containsFuzzy = false;
static ConstructorHelpers::FClassFinder<AFuzzy> FuzzyBlueprint(TEXT("/Game/Blueprints/MyFuzzy"));
if (FuzzyBlueprint.Succeeded())
{
fuzzyBP = FuzzyBlueprint.Class;
}
}
// Called when the game starts or when spawned
void ASpawnpointParent::BeginPlay()
{
Super::BeginPlay();
SetMeshColor();
// Setting the color for the main mesh/the color of the leaf/dirt burst
if (dmiMat)
{
dmiMat->SetVectorParameterValue("SpawnpointColor", meshColor);
}
// Create outline material
overlayMatInstance = UMaterialInstanceDynamic::Create(overlayMat, this);
mesh->SetOverlayMaterial(overlayMatInstance);
// Setting the color for the outline
if(overlayMatInstance)
{
overlayMatInstance->SetVectorParameterValue("Color", offColor);
}
// Getting the value of the arrow rotation for later use
arrowRotation = arrowcomponent->GetComponentRotation();
}
void ASpawnpointParent::SetMeshColor()
{
meshColor = GetRandColor();
// Create mesh material
dmiMat = UMaterialInstanceDynamic::Create(bushMat, this);
mesh->SetMaterial(0, dmiMat);
}
// Called every frame
void ASpawnpointParent::Tick(float DeltaTime)
{
Super::Tick(DeltaTime);
// check current # of fuzzies prepared
// when you capture one, lower the number
// if num < max
// PlaceFuzzies
}
void ASpawnpointParent::FuzzyPrepared()
// For when a fuzzy is hiding in the object but hasn't been spawned yet.
{
// Enable Sound
// https://forums.unrealengine.com/t/how-to-get-a-random-number-in-range-c/721860/3
if (GetWorld()->GetTimerManager().IsTimerActive(TimerHandle))
{
GetWorld()->GetTimerManager().UnPauseTimer(TimerHandle);
}
else
{
GetWorld()->GetTimerManager().SetTimer(TimerHandle, this, &ASpawnpointParent::PlaySound, FMath::RandRange(soundTimeMin, soundTimeMax), true);
}
}
void ASpawnpointParent::ContainsFuzzy()
// CAUTION. NOT used to return whether a spawnpoint contains a Fuzzy. This is used to CHANGE THE VALUE CONTAINS FUZZY = TRUE/FALSE;
{
if (!containsFuzzy)
{
containsFuzzy = true;
FuzzyPrepared();
}
}
FLinearColor ASpawnpointParent::GetRandColor()
// Must be overridden by child class. Cyan is default color.
{
FColor tempColor = FColor::Cyan;
return tempColor;
}
bool ASpawnpointParent::DoesContainFuzzy()
{
if (containsFuzzy)
{
return true;
}
else if (!containsFuzzy)
{
return false;
}
else
{
return false;
}
}
void ASpawnpointParent::PlaySound()
{
if (!playing)
{
// Pick a random Fuzzy sound from the list
// Plays Fuzzy Sound at the location of the root component (raycastcollision)
playing = true;
int index = FMath::RandRange(0, (fuzzySounds.Num()) - 1);
if (fuzzySounds.IsValidIndex(index))
{
UGameplayStatics::PlaySoundAtLocation(this, fuzzySounds[index], GetActorLocation());
}
else
{
GEngine->AddOnScreenDebugMessage(-1, 5.f, FColor::Red, TEXT("Invalid index in FuzzySounds!!"));
}
}
else
{
LeavesBurst(arrowRotation, true);
playing = false;
}
}
void ASpawnpointParent::LeavesBurst(FRotator rotation, bool spawnSound)
{
UNiagaraComponent* particleComp = UNiagaraFunctionLibrary::SpawnSystemAttached(particleBurst, arrowcomponent, NAME_None, FVector(0.f, 0.f, 0.f), rotation, EAttachLocation::KeepRelativeOffset, true);
if (particleComp) {
particleComp->SetNiagaraVariableLinearColor(FString("ParticleColor"), meshColor);
GEngine->AddOnScreenDebugMessage(-1, 5.f, FColor::Red, TEXT("particle spawned"));
// Set a new random time
int index = FMath::RandRange(0, (rustleSounds.Num()) - 1);
if (rustleSounds.IsValidIndex(index) && spawnSound)
{
GEngine->AddOnScreenDebugMessage(-1, 5.f, FColor::Red, TEXT("Playing leaves sounds"));
UGameplayStatics::PlaySoundAtLocation(this, rustleSounds[index], GetActorLocation());
}
else
{
GEngine->AddOnScreenDebugMessage(-1, 5.f, FColor::Red, TEXT("Invalid index in LeavesSounds!!"));
}
}
}
void ASpawnpointParent::OnHit(UPrimitiveComponent* HitComp, AActor* OtherActor, UPrimitiveComponent* OtherComp, FVector NormalImpulse, const FHitResult& Hit)
{
FHitResult hit;
FCollisionQueryParams CollisionParams;
CollisionParams.AddIgnoredActor(this);
if (Hit.bBlockingHit && IsValid(Hit.GetActor()))
{
UInteract_I* Interface = Cast<UInteract_I>(Hit.GetActor());
}
}
// Returns the arrowcomponent's location in world space.
FVector ASpawnpointParent::GetArrowComponentLocation()
{
if (arrowcomponent)
{
FVector location = this->arrowcomponent->GetComponentLocation();
return location;
}
else
{
return FVector(99.f, 99.f, 99.f);
}
}
void ASpawnpointParent::EnableOutline()
{
if (overlayMatInstance)
{
overlayMatInstance->SetScalarParameterValue("Line Thickness", 2.f);
}
else
{
GEngine->AddOnScreenDebugMessage(-1, 5.f, FColor::Red, TEXT("overlayMatInstance null"));
}
}
void ASpawnpointParent::DisableOutline()
{
if (overlayMatInstance)
{
overlayMatInstance->SetScalarParameterValue("Line Thickness", 0.f);
}
}
void ASpawnpointParent::SpawnFuzzy()
{
FVector spawnpointLoc = springArmComponent->GetComponentLocation();
//spawnpointLoc = FVector(spawnpointLoc.X, spawnpointLoc.Y, spawnpointLoc.Z + 300.f);
FActorSpawnParameters spawnParameters;
AFuzzy* fuzzy = GetWorld()->SpawnActor<AFuzzy>(fuzzyBP, spawnpointLoc, FRotator::ZeroRotator, spawnParameters);
if (fuzzy)
{
GEngine->AddOnScreenDebugMessage(-1, 5.f, FColor::Green, "Fuzzy spawned");
containsFuzzy = false;
GetWorld()->GetTimerManager().PauseTimer(TimerHandle);
}
else
{
GEngine->AddOnScreenDebugMessage(-1, 5.f, FColor::Red, "Fuzzy NOT spawned!");
}
}
class UStaticMesh* ASpawnpointParent::GetMeshComponent()
{
return mesh->GetStaticMesh();
}
Environment
I experimented a lot with the environment of this game. While it wasn’t a priority, since gameplay was more important at this stage, I wanted to make something that was visually appealing and fit the theme.
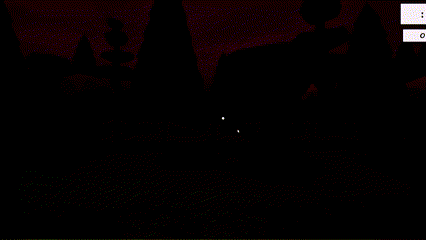
I used a combination of assets I made myself, assets from Itch.io, and assets from the Unreal Engine Marketplace. Inside the editor, I utilized the foliage tool and a custom landscape material to achieve an interesting and effective level. Aside from aesthetics, I aimed to make it clear to the player where the game area was, which I did with fences.
What’s Next?
Since Fuzzy Farmers is a prototype, there is a potentially long way to go for this game. And like many games, this one’s gameplay will most likely dramatically change as development continues!
Bug Fixes
First on the to-do list is bug fixing and other game-breaking or experience-ruining problems. I’ve discovered these through playtesting myself, and having friends and colleagues assist with playtesting.
Priority issues include:
- Fuzzy launch impulse is too great.
- FOV needs to be modified to reduce motion sickness.
- Provide a visual indicator for audio clues.
- Make visual clues more obvious.
- Tree clues burst upwards, which causes the player to frequently miss them.
- E key must be lifted up before the Fuzzy will spawn.
- Menu music is too loud
Gameplay Improvements
After the priority bug fixes have been completed, I can start planning and making changes to the gameplay.
Plans for future development:
- Golden Fuzzy – A new Fuzzy type that is worth 300-500 points (instead of just 100 for the regular Fuzzy). It moves faster, and has smarter AI to dodge the player. It has a gold color and has a particle effect to show it shining.
- Spoiled Fuzzy – A new Fuzzy type that is worth -100 points. While it spawns less frequently, if the player finds this Fuzzy they lose 100 points.
- Fuzzy Collection Method – Currently, the player just presses E to collect a Fuzzy. In future versions, the player will need to capture the Fuzzy in a net. This will make gameplay both more engaging and more challenging.
- Powerups – If you shake a spawnpoint, there is a chance that instead of a Fuzzy being inside, it’s a powerup. This powerup can grant you bonus speed, temporarily slow down time, or enable you to temporarily instantly collect a Fuzzy when it jumps out of a spawnpoint.
- Point system overhaul – Currently, the player earns 100 points per Fuzzy collected. However, in the future, the number of Fuzzies collected in a gameplay session will be added to a total sum of Fuzzies collected. Normal Fuzzies and Golden Fuzzies and any other additional Fuzzies created will have their own counts. As the player collects more Fuzzies and the count increases, they will be able to unlock permanent upgrades.